Firebase Cloud Messaging Android client app, use the FirebaseMessaging API.
Requirements:
1. Android Studio 3.4
2. If you haven't already,add Firebase to your Android project
1.Firebase Register Project Steps:
Step:1.1:
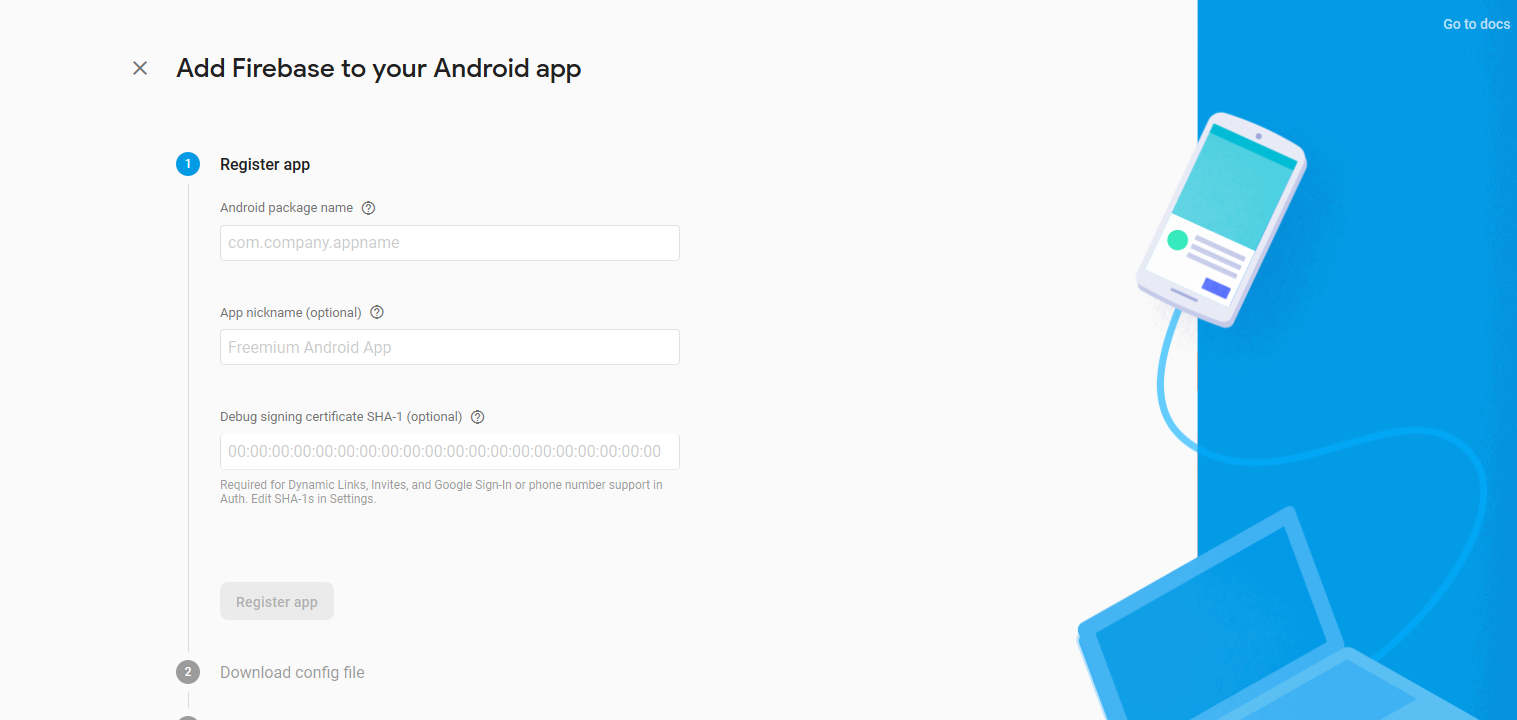
Step:1.2:
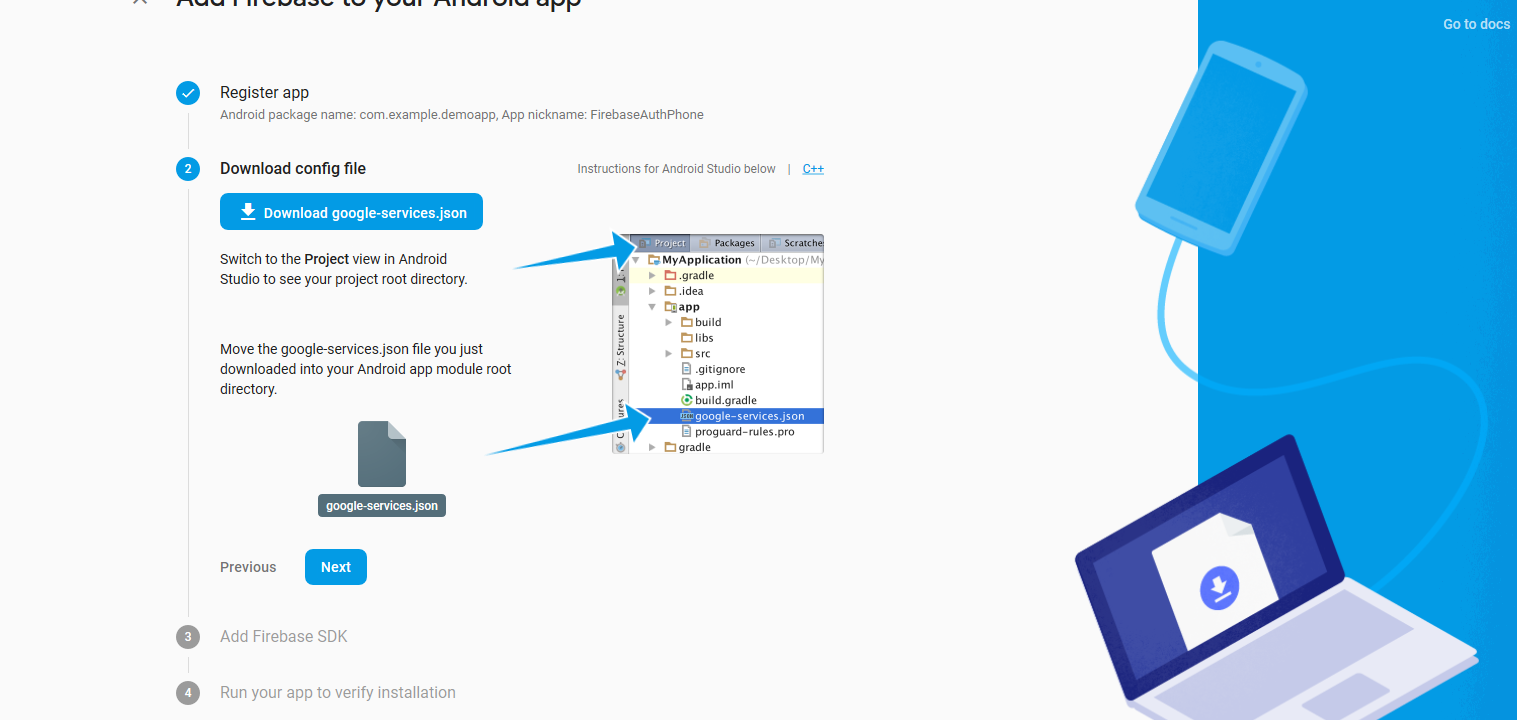
Step:1.3:
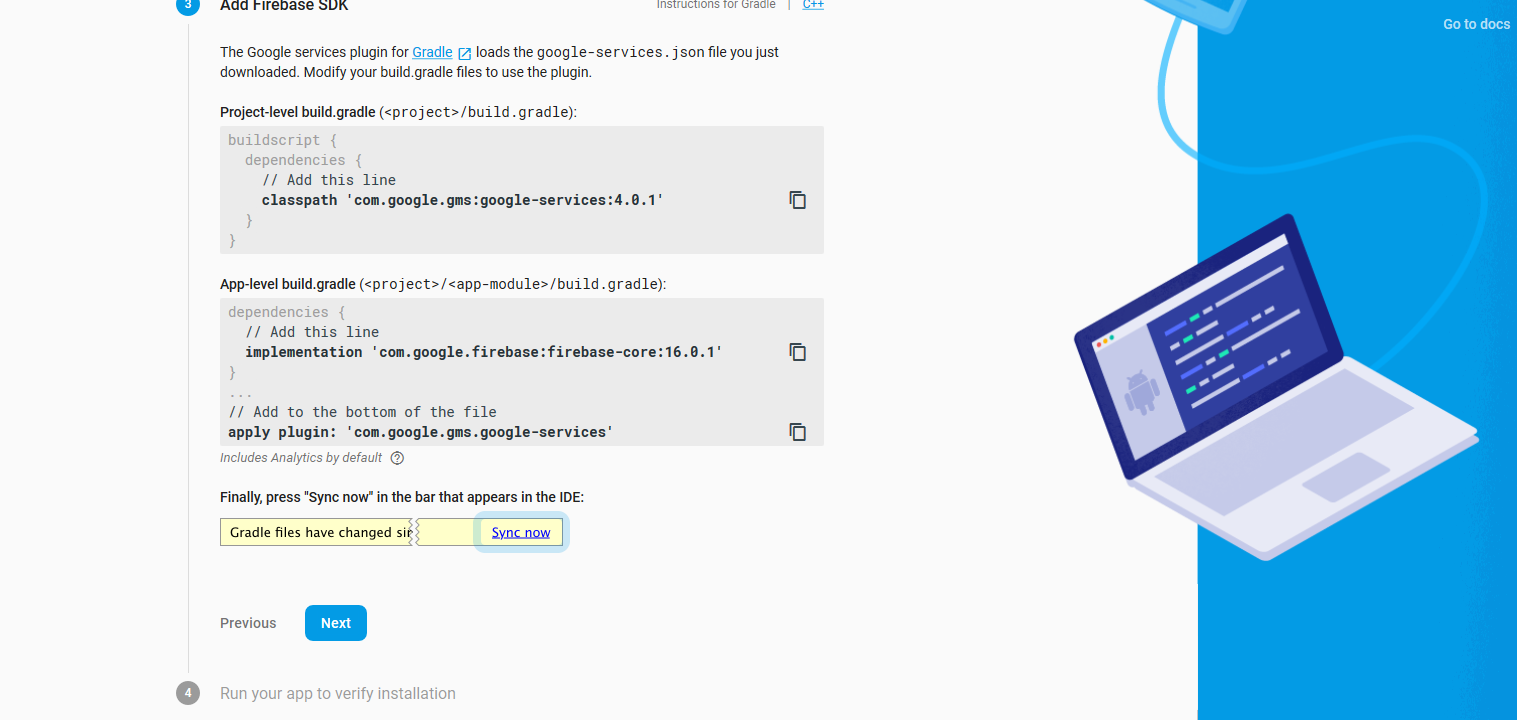
Step:1.4:

2.Set up Firebase and the FCM SDK:
Step:2.1:
In your project-level build.gradle file, make sure to include Google's Maven repository in both your buildscript and allprojects sections.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:3.4.1'
classpath 'com.google.gms:google-services:4.2.0'
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
Step:2.2:
Add the dependency for the Cloud Messaging Android library to your module (app-level) Gradle file (usually app/build.gradle):
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.2'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
//FCM
implementation 'com.google.firebase:firebase-core:17.0.1'
implementation 'com.google.firebase:firebase-messaging:19.0.1'
}
Notifications Overview:
A notification is a message that Android displays outside your app’s UI to provide the user with reminders, communication from other people, or other timely information from your app. Users can tap the notification to open your app or take an action directly from the notification.
3.Edit your app manifest:
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name="com.itw.firebasepushnotificationdemo.MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name=".service.FireBaseMessagingService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
</application>
4.In the Java file, let's start by implementing basic code:
On initial startup of your app, the FCM SDK generates a registration token for the client app instance. If you want to target single devices or create device groups, you’ll need to access this token.
public class FireBaseMessagingService extends FirebaseMessagingService {
private static final String TAG = "FireBaseMessagingService";
public static String NOTIFICATION_CHANNEL_ID = "com.itw.firebasepushnotificationdemo";
public static final int NOTIFICATION_ID = 1;
/**
* Called if InstanceID token is updated. This may occur if the security of
* the previous token had been compromised. Note that this is called when the InstanceID token
* is initially generated so this is where you would retrieve the token.
*/
@Override
public void onNewToken(String token) {
Log.e(TAG, "Refreshed token: " + token);
// If you want to send messages to this application instance or
// manage this apps subscriptions on the server side, send the
// Instance ID token to your app server.
}
/**
* Called when message is received.
*
* @param remoteMessage Object representing the message received from Firebase Cloud Messaging.
*/
// [START receive_message]
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
// [START_EXCLUDE]
// There are two types of messages data messages and notification messages. Data messages
// are handled
// here in onMessageReceived whether the app is in the foreground or background. Data
// messages are the type
// traditionally used with GCM. Notification messages are only received here in
// onMessageReceived when the app
// is in the foreground. When the app is in the background an automatically generated
// notification is displayed.
// When the user taps on the notification they are returned to the app. Messages
// containing both notification
// and data payloads are treated as notification messages. The Firebase console always
// sends notification
// messages. For more see: https://firebase.google.com/docs/cloud-messaging/concept-options
// [END_EXCLUDE]
if (remoteMessage.getData() != null) {
Map data = remoteMessage.getData();
Log.e(TAG, "Message Notification title: " + data.get("title"));
Log.e(TAG, "Message Notification message: " + data.get("message"));
String title = data.get("title"),
message = data.get("message");
showNotification(getBaseContext()title, message);
}
// Also if you intend on generating your own notifications as a result of a received FCM
// message, here is where that should be initiated. See sendNotification method below.
}
// [END receive_message]
private void showNotification(Context context, String title, String message) {
Intent ii;
ii = new Intent(context, MainActivity.class);
ii.setData((Uri.parse("custom://" + System.currentTimeMillis())));
ii.setAction("actionstring" + System.currentTimeMillis());
ii.setFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP | Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pi = PendingIntent.getActivity(context, 0, ii,PendingIntent.FLAG_UPDATE_CURRENT);
Notification notification;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
//Log.e("Notification", "Created in up to orio OS device");
notification = new NotificationCompat.Builder(this,NOTIFICATION_CHANNEL_ID)
.setOngoing(true)
.setSmallIcon(getNotificationIcon())
.setContentText(message)
.setAutoCancel(true)
.setContentIntent(pi)
.setPriority(NotificationCompat.PRIORITY_HIGH)
.setCategory(Notification.CATEGORY_SERVICE)
.setWhen(System.currentTimeMillis())
.setSound(RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION))
.setContentTitle(title).build();
NotificationManager notificationManager = (NotificationManager) context.getSystemService(
Context.NOTIFICATION_SERVICE);
NotificationChannel notificationChannel = new NotificationChannel(NOTIFICATION_CHANNEL_ID,title, NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(notificationChannel);
notificationManager.notify(NOTIFICATION_ID, notification);
}else{
notification = new NotificationCompat.Builder(this)
.setSmallIcon(getNotificationIcon())
.setAutoCancel(true)
.setContentText(message)
.setContentIntent(pi)
.setSound(RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION))
.setContentTitle(title).build();
NotificationManager notificationManager = (NotificationManager) context.getSystemService(
Context.NOTIFICATION_SERVICE);
notificationManager.notify(NOTIFICATION_ID, notification);
}
}
private int getNotificationIcon() {
boolean useWhiteIcon = (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.LOLLIPOP);
return useWhiteIcon ? R.mipmap.ic_notification_icon : R.mipmap.ic_launcher;
}
}
Note: creating notification channel if android version is greater than or equal to oreo